Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the essential features of Python is the ability to print output to the console or terminal. The print statement is a simple yet powerful tool that allows programmers to display output values, messages or debugging information during the program execution. In this Python tutorial, we will explore the print statement, understand its syntax and functionalities, and learn how to use it effectively to print output in various formats and styles. Whether you are a beginner or an advanced programmer, this Python tutorial will help you sharpen your programming skills and improve your code readability.
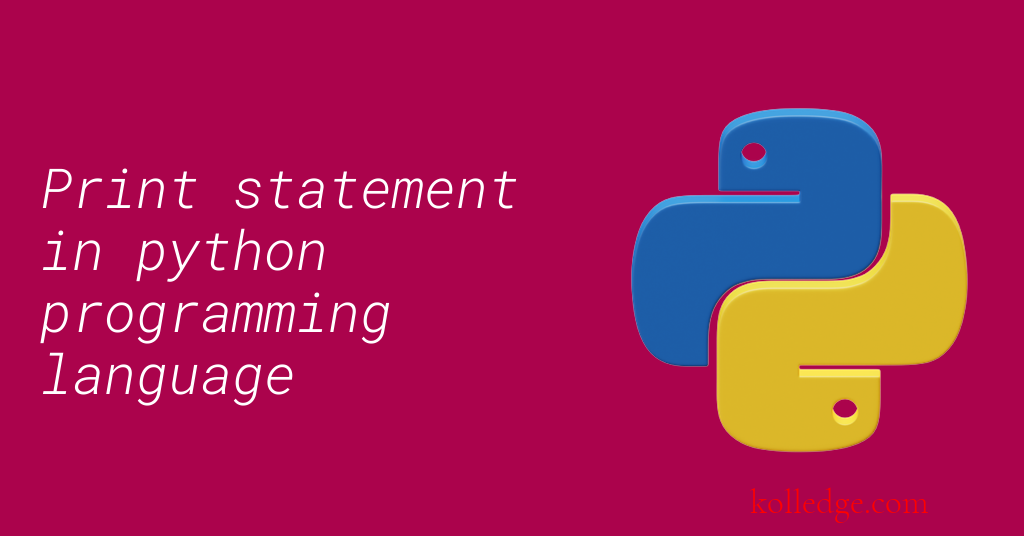
Table of Contents :
- print() statement in python
- Syntax of Python print statement
- Print End Argument
- Print Sep Argument
print() statement in python
- print statement is used for displaying output in python
- print statement in Python programming language is a statement that tells the interpreter to display a value on the screen.
- We can display objects of any data type using the print statement.
Syntax of Python print statement
- The print function takes 5 parameters
- The syntax of print statement is
print (object(s), separator=separator, end=end, file=file, flush=flush)
- The parameters of print are -
- object - value(s) to be printed.
- separator [Optional parameter] - This parameter is used to separate multiple objects in the output.
- end [Optional parameter] - This parameter is used to add specific values like new line "\n", tab "\t". default is “\n”.
- file [Optional parameter] - This parameter is used to set where the values are printed. default is sys.stdout (screen)
- flush [Optional parameter] - This parameter is used to specify if the output is flushed or buffered. Default: False
- The simplest form of print statement is just the word "print" followed by a value in the parentheses:
- Code Sample :
print (3)
# Output
# 3
- We can also use the print statement to print multiple values, separated by commas:
- Code Sample :
print (3, 4, 5)
# Output
# 3 4 5
- To print a string, you must enclose it in quotation marks:
- Code Sample :
print ("Hello, world!")
# Output
# Hello, world!
- We can concatenate two or more strings while printing by using the concatenation operator.
- Code Sample :
print ("Hello " + "world!")
# Output
# Hello world!
- We can also use the print statement to print the results of mathematical expressions:
- Code Sample :
print (3 + 4)
# Output
# 7
- We can use the print statement to print the contents of variables:
- Code Sample :
x = 5
print (x)
# Output
# 5
Print End Argument :
- We can use an optional parameter 'end' within the print statement.
- The end keyword argument specifies what should be printed at the end of the print() function’s output.
- By default, the print() function ends its output with a newline character '\n'.
- We can change this default behavior by specifying a different value for the end keyword argument.
- Specifying an empty string for the end keyword argument will prevent the print() function from adding a newline character to its output:
- Specifying a space character for the end keyword argument will cause the print() function to add a space character to its output instead of a newline character:
- Code Sample :
print("Hello, world!", end=" - ")
print("How do you do?")
# Output
# Hello, world! - How do you do?
# Here the default behvior of print is changed
# By default print will print on new line
# By using " - " character in end statement
# both statements were printed on same line with '-' in between
Print Sep Argument :
- We can use an optional 'sep' argument within the print statement.
- If we need to print multiple items, 'sep' parameter comes in handy.
- 'sep' parameter specifies the characters that will separate the multiple items in the output.
- Code Sample :
print("Hello, world!", "How do you do?", sep=" - ")
# Output
# Hello, world! - How do you do?
# Output
# Here the string given in sep parameter separates the
# two items to be printed.
Prev. Tutorial : Displaying output
Next Tutorial : Output formatting in Python