Beginner Level
Intermediate Level
Advanced Level
Introduction
Python is a popular, high-level programming language used extensively in industries such as web development, data science, and artificial intelligence. One of Python's most important features is its ability to handle different data types. In this tutorial, we will be focusing on the tuples data type in Python. Tuples are an ordered collection of elements that are immutable, meaning they cannot be changed. They are similar to lists, but unlike lists, tuples cannot be modified. This tutorial will cover the basics of tuples, their uses, and how to create and manipulate them in Python.
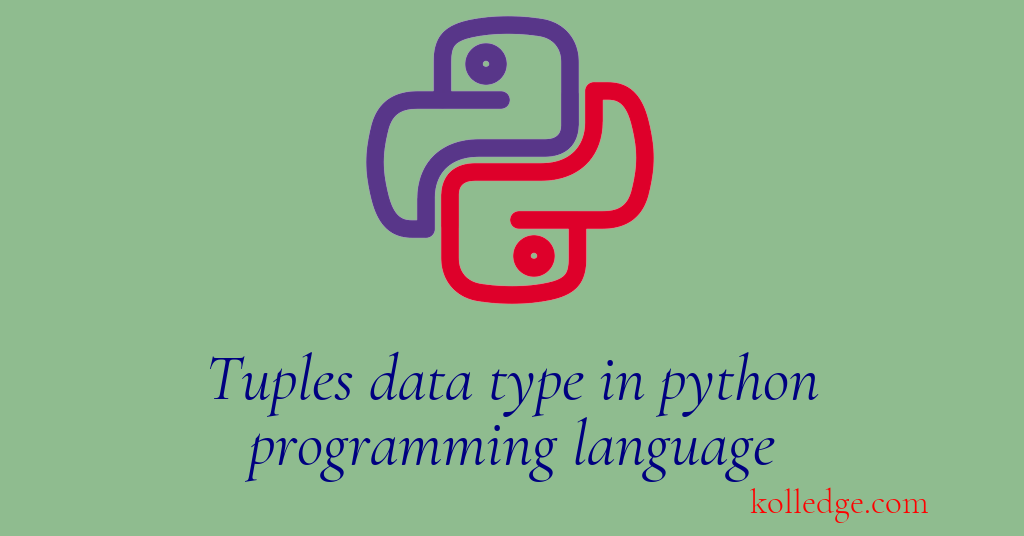
Table of Contents :
- What are Tuples in Python
- Creating tuples using parentheses
- Creating tuples using tuple class
What are Tuples in Python
- Tuples in Python is an ordered collection of elements which is unchangeable.
- In Python programming, a tuple is a data type that represents a sequence of immutable (unchangeable) values.
- The tuple data type is implemented using a
tuple
class in Python. - A tuple is similar to a list, but the main difference is that tuples are immutable (cannot be changed).
- Tuples are used to store data that will not need to be changed, such as a list of employee names, product ID numbers etc.
- Like lists, Tuple maintains the order in which elements were inserted.
- We can create a tuple using the two ways
- Enclosing elements within the parentheses ().
- Creating instance of tuple() class.
- Tuples can contain any type of data, including strings, integers, floats, and even other tuples.
- A tuple can have duplicates elements as well.
- Once a tuple is created, the values inside it cannot be changed.
- For example, suppose we have a tuple
my_tuple (1, 2, 3)
- If we try to reassign one of the values in our tuple above:
my_tuple[0] = 4
We will get an error message saying that "tuples are immutable".
- If we try to reassign one of the values in our tuple above:
- However, we can change the values of a tuple by creating a new tuple with the desired values.
- E.g. if we want to change the value of the first element in our tuple to 4, we can do so by creating a new tuple with the first value set to 4:
my_new_tuple = (4, 2, 3)
- E.g. if we want to change the value of the first element in our tuple to 4, we can do so by creating a new tuple with the first value set to 4:
- Tuples are often used in conjunction with dictionaries, as dictionaries can only store immutable values.
Creating tuples using parentheses :
- We can create tuple variables by enclosing elements within parentheses.
- Code Sample :
t = (1, 2, 3, 4, 5)
print("t is a tuple.")
print(f"Value of t = {t}")
print(f"Type of t is {type(t)}")
# Output
# t is a tuple.
# Value of t = (1, 2, 3, 4, 5)
# Type of t is <class 'tuple'>
Creating tuples using tuple class :
- We can create tuple variables by instantiating tuple class.
- Code Sample :
t = tuple((1, 2, 3, 4, 5))
print("t is a tuple.")
print(f"Value of t = {t}")
print(f"Type of t is {type(t)}")
# Output
# t is a tuple.
# Value of t = (1, 2, 3, 4, 5)
# Type of t is <class 'tuple'>
Prev. Tutorial : List data type
Next Tutorial : Range data type