Beginner Level
Intermediate Level
Advanced Level
Introduction
Time is a crucial aspect in any programming language, and Python offers a built-in module specifically designed for working with time-related tasks. The time
module provides functions that enable you to work with various time-related functions such as parsing dates and times, sleeping, or halting the program for a specific amount of time, measuring execution time, and formatting time. In this tutorial, we will explore how to utilize this module efficiently to handle and manipulate time operations in Python. So, let's dive in!
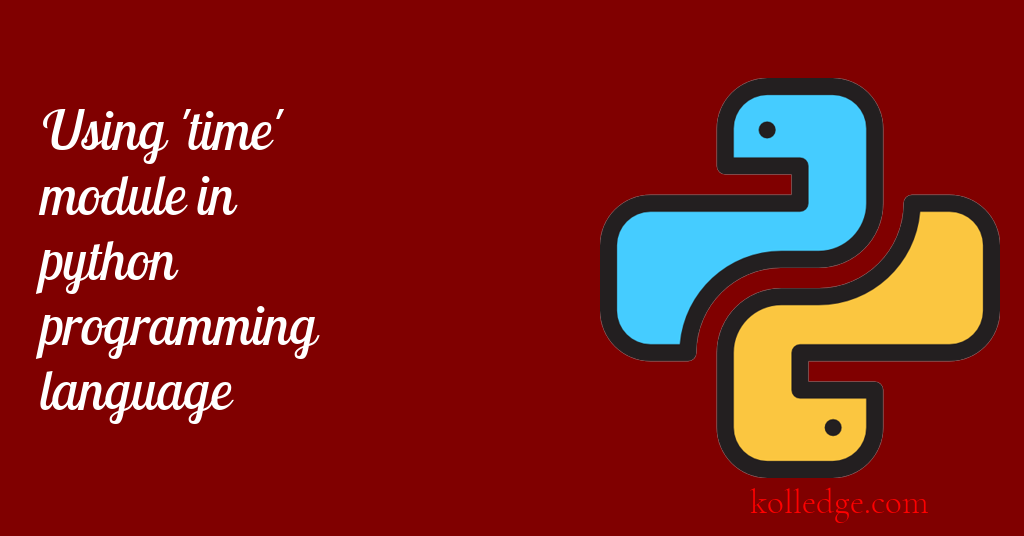
Table of Contents :
- Python time Module
- How to get time string from seconds
- How to delay execution of programs in Python
- How to represent time in a structured way
- Frequently used functions of time Module
- time() function
- ctime() function
- sleep() function
- process_time() function
- localtime() function
- gmtime() function
- mktime() function
- asctime() function
- time.strftime() function
- time.strptime() function
- Format Code List
Python time Module
- Python’s time module has many functions to deal with dates, times, and time intervals.
- other than representing time, the time module can perform other functionalities too like :
- halting the program execution for some time
- measuring how long the code takes to run.
How to get time string from seconds
- We can use the
ctime()
function to convert seconds since epoch to a string. - Epoch is defined as time since January 1, 1970, at 00:00:00 UTC.
- Code Sample :
import time
seconds_since_epoch = 1646882186.9547188
time_string = time.ctime(seconds_since_epoch)
print("Time String:", time_string)
# Output
# Time String: Wed Mar 9 06:49:46 2022
How to delay execution of programs in python
- We can delay the execution of a program for a specified number of seconds using the
sleep()
function. - Code Sample :
import time
print("Executing...")
time.sleep(3)
print("...Delayed Execution")
# Output
# Executing...
# (after 3 seconds) :
# ...Delayed Execution
How to represent time in a structured way
- The
time
module defines thestruct_time
class to represent time in a structured way. - Code Sample :
import time
current_time = time.localtime()
print("Current Time:", current_time)
print("Year:", current_time.tm_year)
# Output:
# Current Time: time.struct_time(tm_year=2022, tm_mon=3, tm_mday=9, tm_hour=7, tm_min=38, tm_sec=54, tm_wday=2, tm_yday=68, tm_isdst=0)
# Year: 2022
Frequently used functions of time Module
- Let's have a look at some commonly used functions of the time module in python :
time() Function
- The
time()
function returns the current time in seconds since epoch. - Epoch is a point in time chosen as a reference point in timekeeping.
- In Unix systems, epoch is defined as January 1, 1970, at 00:00:00 UTC.
- Code Sample :
import time
current_time = time.time()
print("Current Time:", current_time)
# Output
# Current Time: 1646884243.6347806
ctime() Function
- The
ctime()
function takes seconds since epoch as an argument and returns a string representing the corresponding time. - This function is similar to asctime(), but it takes a time in seconds since the Unix epoch instead of a time tuple.
- Code Sample :
import time
current_time = time.time()
time_string = time.ctime(current_time)
print("Time String:", time_string)
# Output
# Time String: Wed Mar 7 07:18:09 2021
sleep() Function
- The
sleep()
function suspends the execution of a program for a given number of seconds. - This function makes our code wait for the given number of seconds.
- It’s useful if we want our code to wait for a certain event to happen, like a button press or a file download etc.
- Code Sample :
import time
print("Executing...")
time.sleep(3)
print("...Delayed Execution")
# Output
# Executing...
# (after 3 seconds) :
# ...Delayed Execution
process_time() Function
- This function returns the sum of the system CPU time and the user CPU time.
- User CPU time is the time taken by the processor to process the code of our application.
- System CPU time is the time taken by the processor to process tasks like accessing the database, taking system input, displaying output, accessing hard disk etc.
- The
process_time()
function does not include the time elapsed due to thesleep()
function. - This function returns the time in float value.
- This function is useful for measuring how long our code takes to run.
- Code Sample :
from time import process_time
n = 500000
# Start timing
start_time = process_time()
sum = 0
for i in range(n):
sum = sum + n ^ 2
# Stop timing
stop_time = process_time()
print("Start time:", start_time)
print("Stop time:", stop_time)
print("Elapsed time during the whole program in seconds:", stop_time - start_time)
# Output
Start time: 0.015625
Stop time: 0.0625
Elapsed time during the whole program in seconds: 0.046875
localtime() Function
- The
localtime()
function returns a structured object representing the local time. - The local time zone is the time zone where the code is running.
- This function takes a time in seconds since the Unix epoch and returns a tuple representing that time in the local time zone.
- Code Sample :
import time
current_time = time.localtime()
print("Current Time:", current_time)
# Output
# Current Time: time.struct_time(tm_year=2020, tm_mon=3, tm_mday=5, tm_hour=7, tm_min=38, tm_sec=54, tm_wday=2, tm_yday=68, tm_isdst=0)
gmtime() Function
- The
gmtime()
function returns a structured object representing the UTC time. - This function takes a time in seconds since the Unix epoch and returns a tuple representing that time in Greenwich Mean Time (GMT).
- GMT is a time zone that’s often used as a reference point for other time zones.
- Code Sample :
import time
current_time = time.gmtime()
print("Current Time in UTC:", current_time)
# Output
# Current Time in UTC: time.struct_time(tm_year=2019, tm_mon=3, tm_mday=9, tm_hour=7, tm_min=38, tm_sec=54, tm_wday=2, tm_yday=68, tm_isdst=0)
mktime() Function
- The
mktime()
function converts a structured object representing local time to seconds since epoch. - This function takes a time tuple in the local time zone and returns the number of seconds since the Unix epoch.
- This is the inverse of
time.localtime()
function. - Code Sample :
import time
current_time = time.localtime()
seconds_since_epoch = time.mktime(current_time)
print("Seconds since Epoch:", seconds_since_epoch)
Output: `Seconds since Epoch: 1646885549.0`
asctime() Function
- The
asctime()
function takes a structured object representing time as an argument and returns a formatted string. - This function takes a time tuple and returns a string representing that time in the local time zone.
- The string is in the format “Tue Aug 13 21:13:48 2019”.
- Code Sample :
import time
current_time = time.localtime()
time_string = time.asctime(current_time)
print("Time String:", time_string)
# Output
# Time String: Mon Mar 9 07:40:53 2018
time.strftime() Function
- The strftime() function converts a tuple or struct_time representing time as returned by
gmtime()
orlocaltime()
to string. - The
time.strftime()
function takes two arguments :- a format string.
- a tuple or struct_time representing time
- The syntax of the function is :
time.strftime(format[, t])
- If the time argument is not provided, the current time as returned by
localtime()
is used. - This function returns a string representing the time in the format specified by the format string.
- The format string contains format codes that will be used to convert the datetime object in desired format.
- These format codes are given in tabular format in the last section of the tutorial.
- Code Sample :
import time
current_time = time.localtime()
time_string = time.strftime("%Y-%m-%d %H:%M:%S", current_time)
print("Time String:", time_string)
# Output
# Time String: 2023-02-09 07:53:07
time.strptime() Function
- The
time.strptime()
function parses a string representing a time according to a format string passed as argument. - The
time.strptime()
function takes two arguments :- a string representing time
- a format string
- The syntax of the function is :
time.strptime(string[, format])
- It returns a
struct_time
- The format string contains format codes that will be used to convert the datetime object in desired format.
- These format codes are given in tabular format in the next section.
- Code Sample :
import time
time_string = "2020-03-22 07:22:07"
current_time = time.strptime(time_string, "%Y-%m-%d %H:%M:%S")
print("Current Time:", current_time)
# Output
# Current Time: time.struct_time(tm_year=2020, tm_mon=3, tm_mday=22, tm_hour=7, tm_min=22, tm_sec=7, tm_wday=6, tm_yday=82, tm_isdst=-1)
Format Code List :
Code | Meaning |
---|---|
%a | Weekday, abbreviated (Sun, Mon, Tue, etc.) |
%A | Weekday, full (Sunday, Monday, Tuesday, etc.) |
%w | Weekday as a decimal number, where 0 is Sunday and 6 is Saturday |
%d | Day of the month, zero-padded (01, 02, ..., 31) |
%b | Month name, abbreviated (Jan, Feb, Mar, etc.) |
%B | Month name, full (January, February, March, etc.) |
%m | Month as a decimal number, zero-padded (01, 02, ..., 12) |
%y | Year without century, zero-padded (00, 01, ..., 99) |
%Y | Year with century, as a decimal number |
%H | Hour in 24-hour format, zero-padded (00, 01, ..., 23) |
%I | Hour in 12-hour format, zero-padded (01, 02, ..., 12) |
%p | AM/PM |
%M | Minute, zero-padded (00, 01, ..., 59) |
%S | Second, zero-padded (00, 01, ..., 59) |
%f | Microsecond, zero-padded (000000, 000001, ..., 999999) |
%z | UTC offset in the form +HHMM or -HHMM |
%Z | time zone name %j - day of the year as a zero-padded decimal number (001-366) |
%U | week number of the year (Sunday as the first day of the week) as a zero padded decimal number (00-53) |
%W | week number of the year (Monday as the first day of the week) as a decimal number (00-53) |
%c | locale's appropriate date and time representation |
%x | locale's appropriate date representation |
%X | locale's appropriate time representation |
%% | a literal '%' character |
Prev. Tutorial : Timedelta
Next Tutorial : Using python time.sleep()