Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the key data structures in Python is a dictionary, which is a collection of key-value pairs. Dictionaries provide an efficient way of storing and retrieving data, and are an essential tool for any Python programmer. In this tutorial, we will explore how to add new key-value pairs to a dictionary in Python. We will cover the basic syntax and usage of the dictionary data type, as well as some useful methods for working with dictionaries.
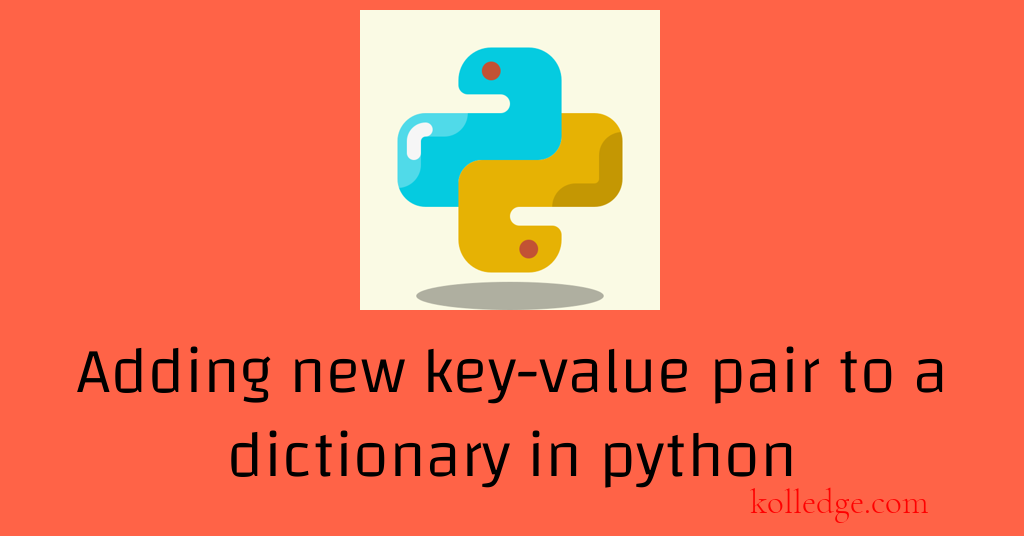
Adding new key-value pairs to a dictionary :
- We can add new key-value pair to the dictionary if the key is not already present in the dictionary
- If the key is already present in the dictionary, the old value of that key will be replaced by the new value.
- The basic syntax of adding new item to the dictionary is :
d[new_key] = new_value
- Code Sample :
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
print("Printing Quantities of fruits :")
print("Quantity of apples = ", quantities.get("apple"))
print("Quantity of oranges = ", quantities.get("oranges"))
print("Quantity of banana = ", quantities.get("banana"))
print("Quantity of Mangoes = ", quantities.get("Mangoes"))
# Adding new key-value pair
quantities["Guavas"] = 50
print("Quantity of Guavas = ", quantities.get("Guavas"))
# Output
# Printing Quantities of fruits :
# Quantity of apples = 10
# Quantity of oranges = 20
# Quantity of banana = 25
# Quantity of Mangoes = 35
# Quantity of Guavas = 50
Prev. Tutorial : Accessing values of a dict
Next Tutorial : Modify/delete dict entry