Beginner Level
Intermediate Level
Advanced Level
Introduction
SQL (Structured Query Language) is a standard language used for managing relational databases. In this tutorial, we will explore how to use SQL SELECT queries in Python programming. With the help of Python's built-in libraries, we can connect to a database, execute SQL SELECT queries, and retrieve desired data from the database. This tutorial will cover the fundamental concepts of SQL SELECT queries and demonstrate how to use them in Python. By the end of this tutorial, you will have a strong understanding of how to integrate SQL into your Python code for efficient data manipulation and retrieval.
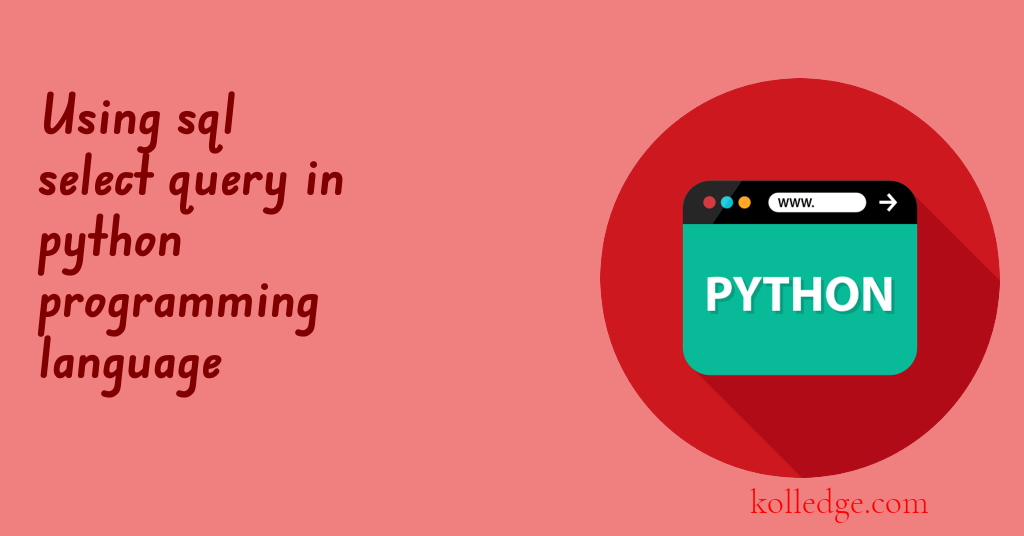
Table of Contents :
- How to Execute select query in MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: Execute select query
How to Execute select query in MySQL with Python :
- Follow the steps given below to Execute select query in MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: Execute select query
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using pip through the command line.
- Code Sample :
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To create a new database in MySQL using Python, we must have a connection to the MySQL server.
- To connect to a MySQL server in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- We need to pass the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- user : username for the MySQL account
- password : password for the MySQL account database
- database : The name of the database to connect to
- To study package specific code for connecting to MySQL read our tutorial on connecting to MySQL server.
Step 3: Create a Cursor Object
- After establishing a connection to the MySQL server, your next step is to create a cursor object.
- This is done using the cursor() method provided by mysql.connector or PyMySQL.
- Code Sample :
cursor = mydb.cursor()
Step 4: Execute select query
- To retrieve data from a MySQL table using Python, use the `SELECT` statement.
- Use the `execute()` method on the cursor object to execute the query.
- Use the `fetchall()` method to retrieve all rows returned from the query.
- Code Sample :
query = "SELECT * FROM employees"
cursor.execute(query)
result = cursor.fetchall()
for row in result:
print(row)
Prev. Tutorial : Insert values in mysql table
Next Tutorial : Using sql where clause