Beginner Level
Intermediate Level
Advanced Level
Introduction
In this tutorial, we will focus on utilizing SQL join clause in Python programming language. We will start by introducing the various kinds of SQL join clause available and their functions. We will then provide step-by-step instructions on how to implement SQL join clause in Python. This tutorial will equip learners with the necessary skills to manipulate and analyze data from multiple tables in Python, making it a valuable resource for any data enthusiast or programmer.
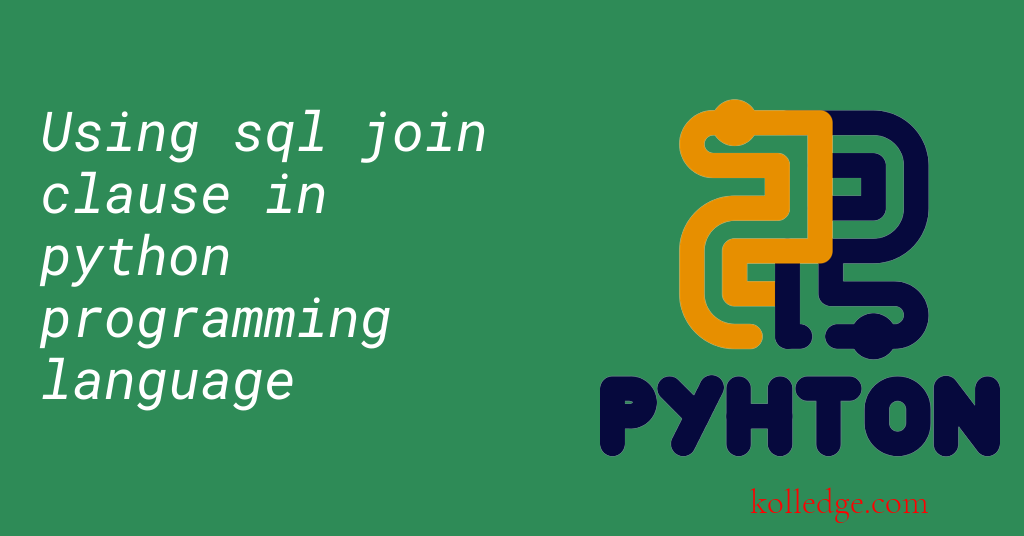
Table of Contents :
- What is SQL Join clause
- Different types of SQL joins
- Inner Join
- Outer Join
- Left Join
- Right Join
- How to use sql join clause in MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use sql join clause
What is SQL Join clause :
- SQL Join Clause is used to combine data from two or more tables based on a common column.
- The common column is called the join column.
- The data from each table is combined into a single row.
Different types of SQL joins :
There are four types of SQL Joins:
- INNER JOIN: The INNER JOIN clause returns all rows from both tables that satisfy the join condition.
- OUTER JOIN: The OUTER JOIN clause returns all rows from both tables, even if they don't satisfy the join condition.
- LEFT JOIN: The LEFT JOIN clause returns all rows from the left table, even if they don't satisfy the join condition.
- RIGHT JOIN: The RIGHT JOIN clause returns all rows from the right table, even if they don't satisfy the join condition.
- Python programming language supports all these types of SQL Joins.
How to use sql join clause in MySQL with Python :
- Follow the steps given below to use sql join clause in MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use sql join clause
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using
pip
through the command line. - Code Sample :
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To create a new database in MySQL using Python, we must have a connection to the MySQL server.
- To connect to a MySQL server in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- We need to pass the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- user : username for the MySQL account
- password : password for the MySQL account database
- database : The name of the database to connect to
- To study package specific code for connecting to MySQL read our tutorial on connecting to MySQL server.
Step 3: Create a Cursor Object
- After establishing a connection to the MySQL server, your next step is to create a cursor object.
- This is done using the
cursor()
method provided by mysql.connector or PyMySQL. - Code Sample :
cursor = mydb.cursor()
Step 4: use sql join clause
- To join two or more tables in a MySQL database using Python, use the
JOIN
clause in yourSELECT
statement. - Use the
execute()
method on the cursor object to execute the query. - Code Sample :
query = "SELECT employees.name, departments.department_name FROM employees JOIN departments ON employees.department_id = departments.id"
cursor.execute(query)
result = cursor.fetchall()
for row in result:
print(row)
Prev. Tutorial : Using sql limit clause
Next Tutorial : Processes and Threads