Introduction
In Python, class methods, static methods, and instance methods are three types of methods that are used to define the behavior of classes and objects. Understanding the differences between these methods is essential for building efficient and effective applications in Python. In this tutorial, we’ll explore the differences between these three types of methods in Python.
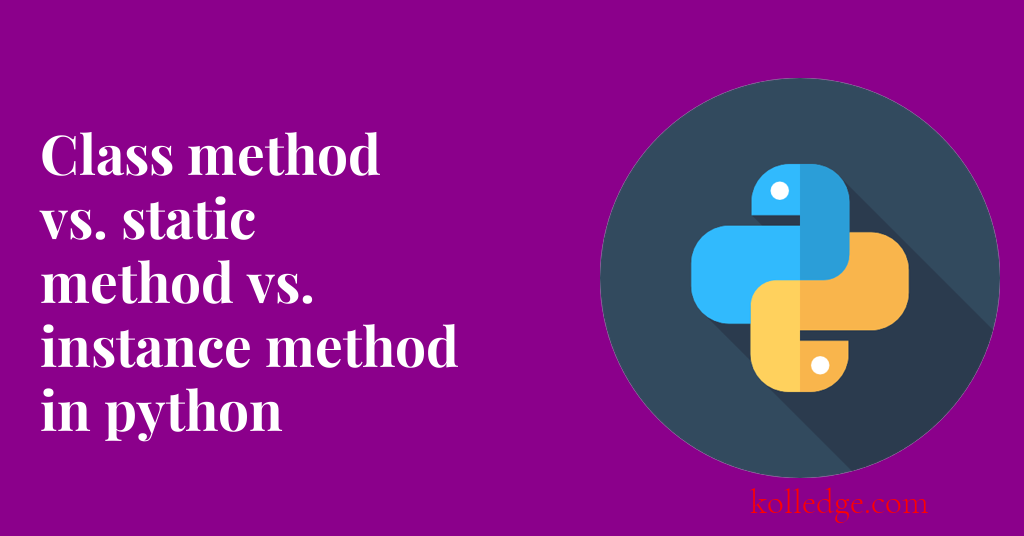
Table of Contents :
- Difference in Primary Use
- Difference in Method Definition
- Difference in Method Call
- Difference in Attribute Access
- Difference in Class Bound and Instance Bound
This tutorial is a just a gist of what we have read so far about class methods, instance methods and static methods. This tutorial is aimed to be used as a revision point or reference point. We have detailed tutorials with code samples on each of these topics. The points jotted down in upcoming sections are discussed in detail in those tutorials. It is recommended that readers go through the detailed tutorials mentioned below before using this tutorial as a reference point :
Difference in Primary Use:
- Class method : Used to modify class-specific properties and return class-specific values.
- Static method : Utility methods used to perform operations that are common to the class and its instances.
- Instance method : Used to work with instance-specific data and perform operations that are unique to each instance.
Difference in Method Definition
- Class method : Defined using the
classmethod
decorator, takescls
as its first parameter which refers to the class and can modify class variables. - Static method : Defined using the
staticmethod
decorator, takes no special first parameter, and cannot modify anything about the class or object state. - Instance method : Defined as a regular method with
self
as the first parameter and can modify instance variables.
Difference in Method Call:
- Class method : Can be called both on the class and its instances, but the first argument must always be the class.
- Static method : Can be called both on the class and its instances, with no argument explicitly passed for the first parameter.
- Instance method : Can only be called on the instance.
Difference in Attribute Access:
- Class method : Can access and modify only class-level data, cannot access instance-level data.
- Static method : Cannot access or modify the class or instance data as it does not take class or instance as first argument. If we want to access or modify class data within static method, we can do it using the class name explicitly. But if such need arises, it is better to use a class method instead of static method.
- Instance method : Can access and modify both instance-level data and class-level data.
Difference in Class Bound and Instance Bound:
- Class method : Bound to the class, not the instance.
- Static method : Neither bound to the class nor instance.
- Instance method : Bound to the instance, not the class.
Here are some examples of how these methods are defined and used:
class Employee:
company_name = "ABC Inc."
def __init__(self, emp_name, emp_id, emp_desig):
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
@staticmethod
def show_company(cmp_name):
print(f"The name of the company is : {cmp_name}")
@classmethod
def change_cmp(cls):
cls.company_name = "XYZ Inc."
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
emp_1 = Employee("Steve", "k2591", "Manager")
# Calling instance method
emp_1.print_details()
# Calling the static method
cmp_name = Employee.company_name
Employee.show_company(cmp_name)
# Calling a class method
Employee.change_cmp()
# Calling the static method again
cmp_name = Employee.company_name
Employee.show_company(cmp_name)
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# The name of the company is : ABC Inc.
# The name of the company is : XYZ Inc.
Prev. Tutorial : Static methods
Next Tutorial : Encapsulation