Beginner Level
Intermediate Level
Advanced Level
Introduction
If..else statements are a fundamental concept in programming and are used to make decisions based on conditions. With If..else statements, you can tell your program to execute certain code if a condition is met, and execute different code if the condition is not met. This tutorial is designed for those with basic knowledge of Python who want to expand their understanding of If..else statements. By the end of this tutorial, you will be well equipped to write If..else statements to improve the functionality of your Python programs. Let's get started!
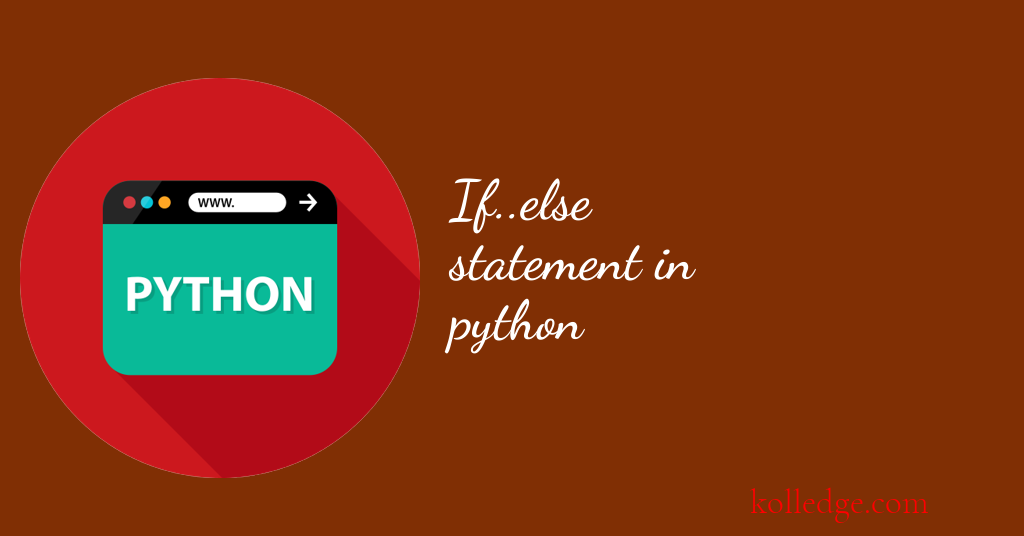
Table of Contents :
- Python If..else Statement
- Control flow for if....else statement
Python If..else Statement
- While writing a code usually we need to perform one task when a logical condition is True and another task when the logical condition is False.
- This functionality is implemented using the if...else statement.
- The syntax of the if...else statement in Python is:
if Logical_Condition:
code-block-If
else:
code-block-Else
outer-code-block
Control flow for if....else statement :
- The Logical condition of the if statement is evaluated.
- If the logical condition is True the statements in the code-block-If are executed.
- If the logical condition is False the statements in the code-block-If are ignored and the statements in the code-block-Else are executed.
- The control flow then comes to the outer-code-block and its statements are executed.
- No matter the condition of the if statement is True or False - the statements in the outer-code-block are always executed.
- Code Sample : When the logical condition of if statement evaluates to True.
x = 10
if x > 5:
print("I am the code-block-If")
print("Logical condition is True")
print()
else:
print("I am the code-block-Else")
print("Logical condition is False")
print()
print("I am the outer-code-block.")
# Output
# I am the code-block-If
# Logical condition is True
#
# I am the outer-code-block.
- Code Sample : When the logical condition of if statement evaluates to False.
x = 2
if x > 5:
print("I am the code-block-If")
print("Logical condition is True")
print()
else:
print("I am the code-block-Else")
print("Logical condition is False")
print()
print("I am the outer-code-block.")
# Output
# I am the code-block-Else
# Logical condition is False
#
# I am the outer-code-block.
Prev. Tutorial : If statement
Next Tutorial : If…elif…else Statement