Introduction
Comments are a crucial component of any programming language, including Python. These are lines of text within a Python program that are not executed as part of the code, but serve as explanatory notes for anyone reading the code. Commenting your code in Python makes it easier for other programmers to follow how it works, and also helps you understand your own code when you revisit it later. In this tutorial, we will take an in-depth look at comments in Python programming language. We will cover the basics of commenting syntax, the various ways to add comments in your code, and best practices for commenting in Python. By the end of this tutorial, you’ll have the tools you need to write clear, concise, and effective comments in your Python programs.
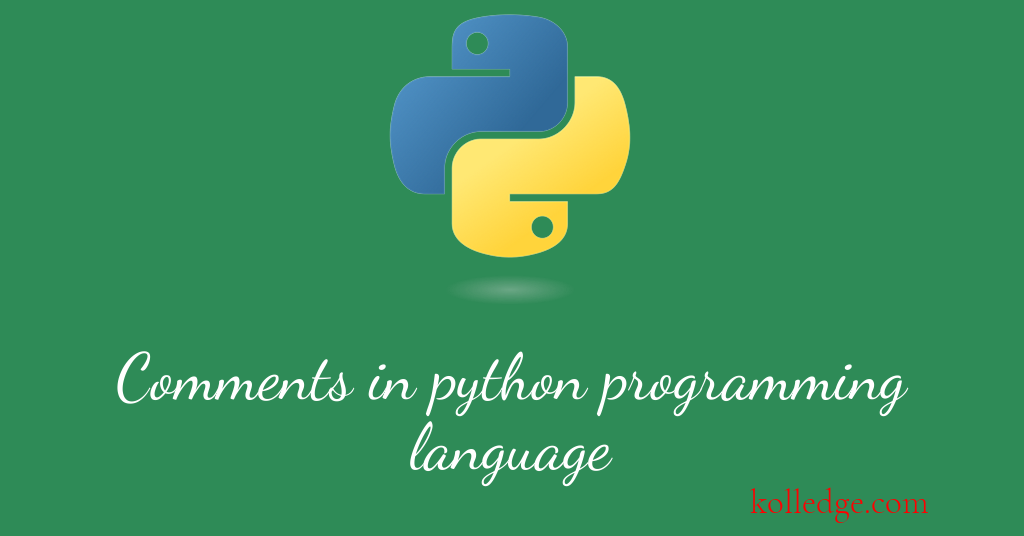
Table of Contents :
- What are Comments in a Programming Language
- Why should we add comments in our code
- Best Practices for adding comments
- Different ways of adding comments in Python
- single line Comments
- Multi line comments
- Inline comments
- block comments
- docstring comments
What are Comments in a Programming Language
- Comments are lines that are not executed as part of the code.
- They are used to explain what the code does and to make the code easier to read.
- In Python comments can be added to the code by using the "#" symbol.
- Anything after the "#" symbol on a line will be ignored by the Python interpreter.
- For example, in our first python program we printed "Hello, world!" to the console:
print("Hello, Kolledge scholars!!")
- We can add a comment to this code like this:
# This code prints "Hello, Kolledge scholars!!" to the console
print("Hello, Kolledge scholars!!")
Using Comments To disable Code :
- Comments can also be used to disable code. This can be useful if you are testing different pieces of code and want to temporarily disable a certain section.
- To disable a section of code, simply add a "#" symbol in front of each line.
- For example, the following code will print "Hello, world!" to the console:
print("Hello, Kolledge scholars!!")
print("Hello Again!!!")
# Output
# Hello, Kolledge scholars!!
# Hello Again!!!
- Adding a comment to this code would look like this:
# print("Hello, Kolledge scholars!!")
print("Hello Again!!!")
# Output
# Hello Again!!!
- Now this code will print only the second line to the console because the first line has been commented out.
Why should we add comments in our code
- It is a good practice to write comments to explain complicated parts of our code.
- comments help others to understand our code and logic better.
- Also sometimes if we revisit our own code after a long time, we tend to forget the exact logic and approach that we have coded.
- Hence comments help us understand our own code as well.
Best Practices for adding comments
- We should try and keep our comments very to the point.
- Our comments should always make sense.
- Every comment in our code should add some value to our code.
- comments should be written only when needed and not just for the sake of commenting.
- If the code is self-explanatory we should avoid writing comments for it.
- E.g. The comments shown below are needless as the code is self explanatory.
# defining a variable named index
# assigning a value of 10 to variable index
index = 10
Different ways of adding comments in Python :
In Python we can add comments in the following ways as per our needs -
- single line Comments
- Multi line comments
- Inline comments
- block comments
- docstring comments
Single-line comments in Python
- A hash sign (#) is used to add a single line comment in Python.
- Anything written after the hash sign is ignored by the interpreter.
- A single line comment is effective till the end of the line.
- Usually these comments are added to explain what our code is doing.
- Code Sample :
# This is a single line comment
print("Hello, Kolledge scholars!!")
# Output
# Hello, Kolledge scholars!!
Multi - line Comments in Python
- There is no discrete way of adding multi-line comments in Python.
- To add multi line comments we need to simply add hash sign to each line separately.
- Multi-line strings can also be used to add multi line comments.
- A string literal enclosed within triple quotes is called multi-line string.
- Python just ignores any string literal that is not assigned to any variable.
- Hence we can create a multi-line String literal without assigning it to any variable. This can be used as a comment
- Code Sample :
'''
This is a Multi-line String literal.
This can be used as a multi-line comment
because Python interpreter ignores any
multi-line string that is not assigned to a variable.
'''
print("Hello, Kolledge scholars!!")
# Output
# Hello, Kolledge scholars!!
Inline comments or trailing comments in Python
- These are the comments added on the same line as the code itself.
- These comments also start with a hash sign.
- Trailing comments should be separated from the main code by at-least two spaces for better readability.
- Inline comments are used to explain the code statement in a precise manner.
- Code Sample :
print("Hello, Kolledge scholars!!") # This is an inline comment
# Output
# Hello, Kolledge scholars!!
Block Comments in Python
- Block comments are used to describe functions, classes and files.
- Block comments are added at the beginning of files.
- Block comments are added before functions.
- Code Sample :
# Returns a customized message to the scholar
# parameter 1 - scholar_name : Name of the Kolledge scholar
# parameter 2 - msg_type : 1 for simple message, 2 for funky message
# returns - Customized message string
def welcome(scholar_name, msg_type):
if msg_type == 1:
msg = "Hello " + scholar_name
elif msg_type == 2:
msg = "Howdy " + scholar_name + "!!! Yo."
return msg
Docstring comments in Python
- Docstring comments are used to describe
- some conventions for docstring comments
- These comments are added just after the declaration
- They can be single or multi-line comments.
- Docstring begins with a capital letter.
- Docstring ends with a period.
- Code Sample :
def calc_area(radius):
"""Calculates the area of a circle as per the value of radius param."""
return math.pi * radius ** 2
Prev. Tutorial : Namespaces
Next Tutorial : Variables