Beginner Level
Intermediate Level
Advanced Level
Introduction
Bytes and ByteArray are binary data types that allow for the storage and manipulation of binary data. These data types play a critical role in many aspects of programming, including cryptography, networking, and data serialization. This tutorial will cover the basics of Bytes and ByteArray data types in Python, their differences, and how to use them effectively in your code.
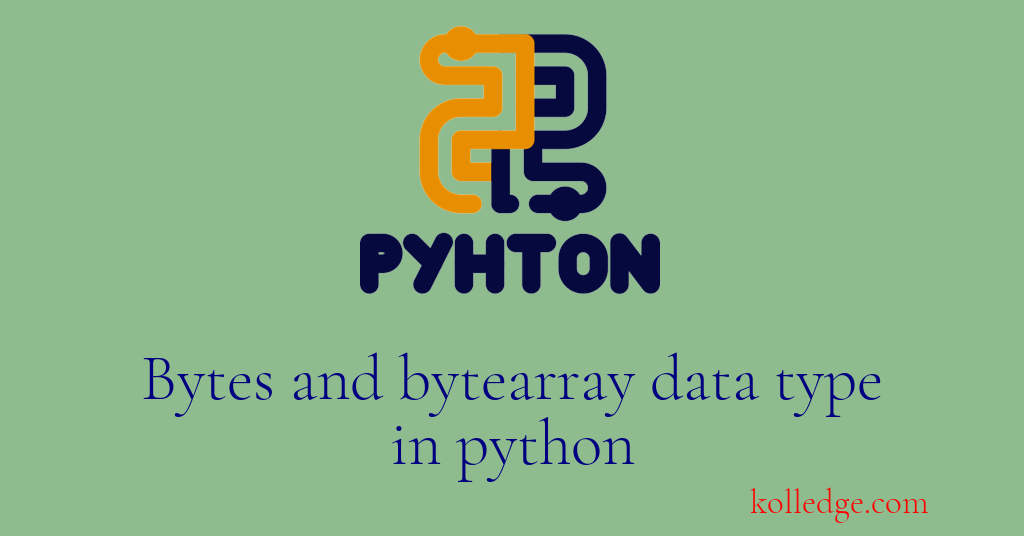
Table of Contents :
- Bytes data type in Python
- Bytearray data type in Python
Bytes data type in Python
- Bytes is a data type that consists of 8 bits.
- Bytes data type is used for working with Binary data.
- Bytes data type comes in handy when we are dealing with images, videos, and audio files.
- Bytes objects are actually an immutable sequence of single bytes.
- Bytes literals has the same syntax as that for string literals, the only difference being that a
b
prefix is added in case of Bytes literals. - The allowed values in bytes data type are
0 to 255
. - Python interpreter returns a value error if we try to use values outside this range for bytes data type.
- Bytes type can be created by instantiating the
bytes
class. - Code sample :
b = bytes(3)
print(f"Value of b = {b}")
print(f"Type of b is {type(b)}")
# Output
# Value of b = b'\x00\x00\x00'
# Type of b is <class 'bytes'>
Bytearray data type in Python
- The bytearray data type behaves similar to the bytes data type, the only difference being that bytearray is mutable.
- bytearray object can be created instantiating the
bytearray
class. - Code sample :
b = bytearray(3)
print(f"Value of b = {b}")
print(f"Type of b is {type(b)}")
# Output
# Value of b = bytearray(b'\x00\x00\x00')
# Type of b is <class 'bytearray'>
Prev. Tutorial : Sets data type
Next Tutorial : Memoryview data type