Introduction
In programming, loops are an essential concept that allows us to automate repetitive tasks. A while loop, in particular, is a control flow statement that allows a block of code to be executed repeatedly while a specified condition remains true. While loops make it easy to write programs that need to perform a certain operation over and over again until a certain condition is met. In this tutorial, we’ll explore the basics of while loops in Python, how to construct them, and some best practices for using them in your code. By the end of this tutorial, you’ll have a solid understanding of how to use while loops in Python programming.
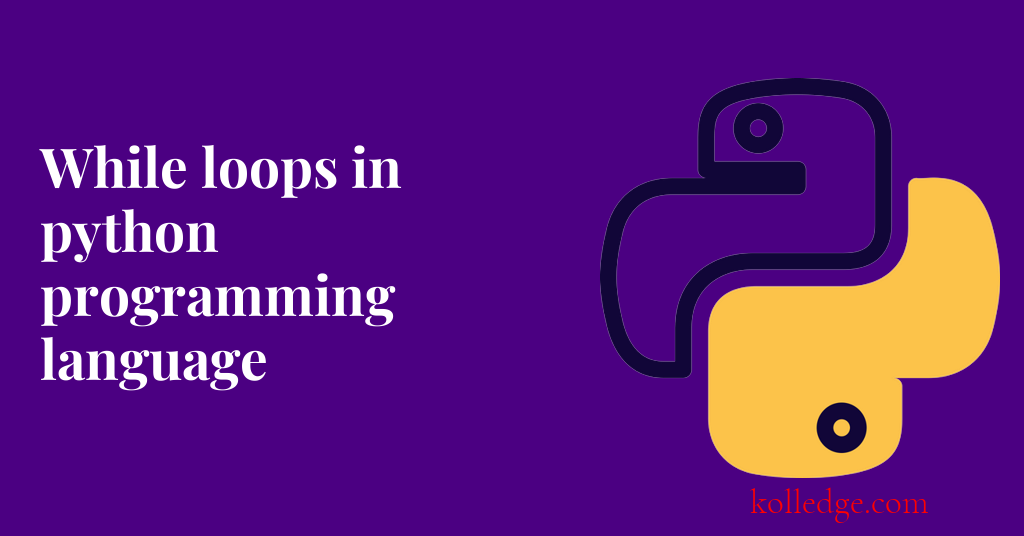
Table of Contents :
- While loop in Python
- Control flow of while loop
- Important Points for using while loop
While loop in Python
- While loop is a technique that can be used to execute a code-block over and over again.
- While statement has a logical condition part.
- The condition is evaluated before every iteration.
- The code block of the while loop is executed as long as the logical condition remains true.
- The syntax of the while loop is as follows -
while logical-condition:
While-code-block
Control flow of while loop -
- Python interpreter evaluates the logical condition of the while loop before every iteration.
- If the condition is true the while - code-block is executed.
- If the condition is false the control jumps out of the while loop.
- The code block is executed repeatedly as long as the condition evaluates to true.
- Code Sample :
index = 1
while index < 5:
sqr = index ** 2
print(f"Value of index = {index}")
print(f"Square of index = {sqr}")
print()
index += 1
print("Coming out of while loop")
print(f"Value of index = {index}")
# Output
# Value of index = 1
# Square of index = 1
# Value of index = 2
# Square of index = 4
# Value of index = 3
# Square of index = 9
# Value of index = 4
# Square of index = 16
# Coming out of while loop
# Value of index = 5
Important Points for using while loop
- care Should be taken that there is some logic within the code_block that makes the condition false so that the execution of the while loop Stops.
- If the condition of while loop never evaluates to false the while loop will run forever.
- This type of loop is called infinite loop.
- If the condition of the while loop is false right from the beginning, the body of the while loop is not executed even once and the while loop doesn't do anything.
Prev. Tutorial : For loop
Next Tutorial : For...else loop