Beginner Level
Intermediate Level
Advanced Level
Introduction
Strings are fundamental data types in programming, and learning to manipulate them is a crucial skill for any programmer. One common operation you might have to perform is reversing a string, but it's not always as straightforward as it seems. Fortunately, Python offers several ways to reverse a string efficiently, whether you're working with a single word or a multi-line text. By the end of this tutorial, you'll have a thorough understanding of how to reverse a string in Python, and you'll be equipped with the tools to tackle similar string-manipulating problems in the future.
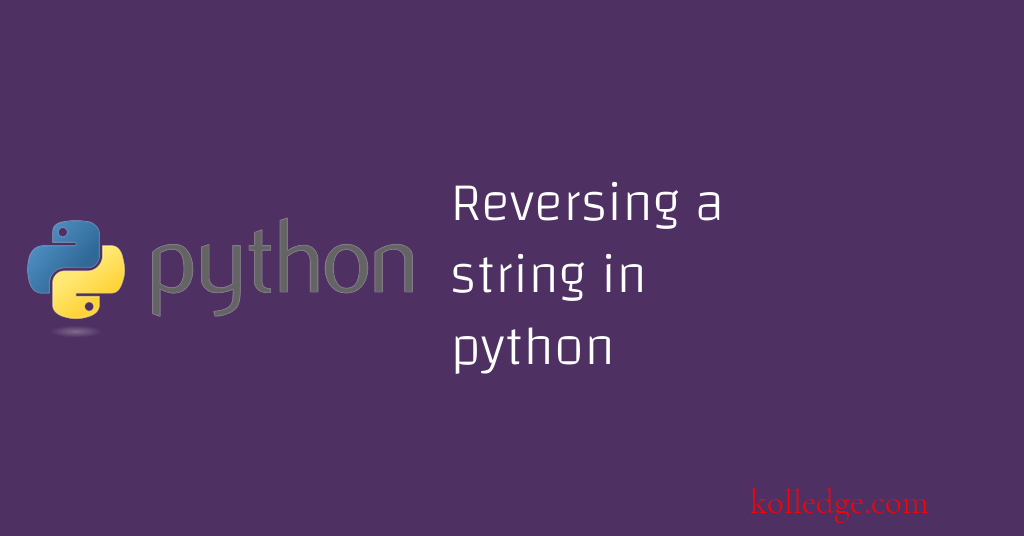
Table of Contents :
- Different Ways of reversing a string in Python :
- Reverse a string in Python using a loop
- Reverse a string in Python using recursion
- Reverse a string in Python using stack
- Reverse a string in Python using an extended slice
- Reverse a string in Python using reversed() method
- Reverse a string in Python using list comprehension
- Reverse a string in Python using join function
Different Ways of reversing a string in Python :
- In Python we can reverse a string using different methods, these include :
- using a loop
- using recursion
- using stack
- using an extended slice
- using reversed() method
- using list comprehension
- using join function
- We'll discuss each of these methods in the upcoming sections.
Reverse a string in Python using a loop:
- We can use a for loop or a while loop to iterate through the string in a reverse manner.
- Concatenate each character to a new string variable.
- Code Sample :
my_string = "Hello, world!"
reversed_string = ""
for i in range(len(my_string) - 1, -1, -1):
reversed_string += my_string[i]
print(reversed_string)
# Output
# !dlrow ,olleH
Reverse a string in Python using recursion:
- Recursion is a powerful programming technique to break down complicated problems into smaller ones.
- We can define a recursive function that slices a portion of the string and concatenates each character to a reversed sub-string.
- Return the reversed sub-string when the original string is sliced completely.
- Code Sample :
def reverse_string(string):
if len(string) == 0:
return string
else:
return reverse_string(string[1:]) + string[0]
my_string = "Hello, world!"
print(reverse_string(my_string))
# Output
# !dlrow ,olleH
Reverse string in Python using stack:
- Convert the string to a list or array, and use a stack data structure to push each character in reverse order.
- Pop each character from the stack and concatenate them to a new string variable.
- Code Sample :
my_string = "Hello, world!"
stack = []
for character in my_string:
stack.append(character)
reversed_string = ""
# Popping characters one by one from stack
while len(stack) > 0:
reversed_string += stack.pop()
print(reversed_string)
# Output
# !dlrow ,olleH
Reverse string in Python using an extended slice:
- We can use an extended slice syntax to reverse the string in one line of code.
- Code Sample :
my_string = "Hello, world!"
reversed_string = my_string[::-1]
print(reversed_string)
# Output
# !dlrow ,olleH
Reverse string in Python using reversed() method :
- We can use the
reversed()
method to reverse a string. - This method returns a reversed iterator, which can be joined or converted to a string.
- Code Sample :
my_string = "Hello, world!"
reversed_string = ''.join(reversed(my_string))
print(reversed_string)
# Output
# !dlrow ,olleH
Reverse string in Python using list comprehension :
- We can use list comprehension to reverse a string by
- creating a list of the string's characters in reverse order.
- using the
join()
method to join the list elements to create a string.
- Code Sample :
my_string = "Hello, world!"
reversed_string = ''.join([my_string[index] for index in range(len(my_string) - 1, -1, -1)])
print(reversed_string)
# Output
# !dlrow ,olleH
Reverse string in Python using join function :
- We can make use of string methods to reverse a string.
- We can use the built-in
reversed()
function to reverse a string by creating a list of the string's characters in reverse order. - then use the
join()
function to Join the list elements to create a string. - Code Sample :
my_string = "Hello, world!"
reversed_string = ''.join(reversed(list(my_string)))
print(reversed_string)
# Output
# !dlrow ,olleH
Prev. Tutorial : Looping through a string
Next Tutorial : Slicing a string