Introduction
Variables form the backbone of any programming language, as they help store data values and manipulate them to perform calculations, comparisons, and other operations. In Python, variables are dynamically-typed, meaning that they don't require explicit typing before use, and can change types as needed during runtime. Understanding how to define, assign, and use variables is essential in Python programming and will equip you to solve various computing problems effectively. In this tutorial, you will learn about the basics of variables in Python, including syntax, data types, and best practices for variable naming and use in programming. Let's dive in!
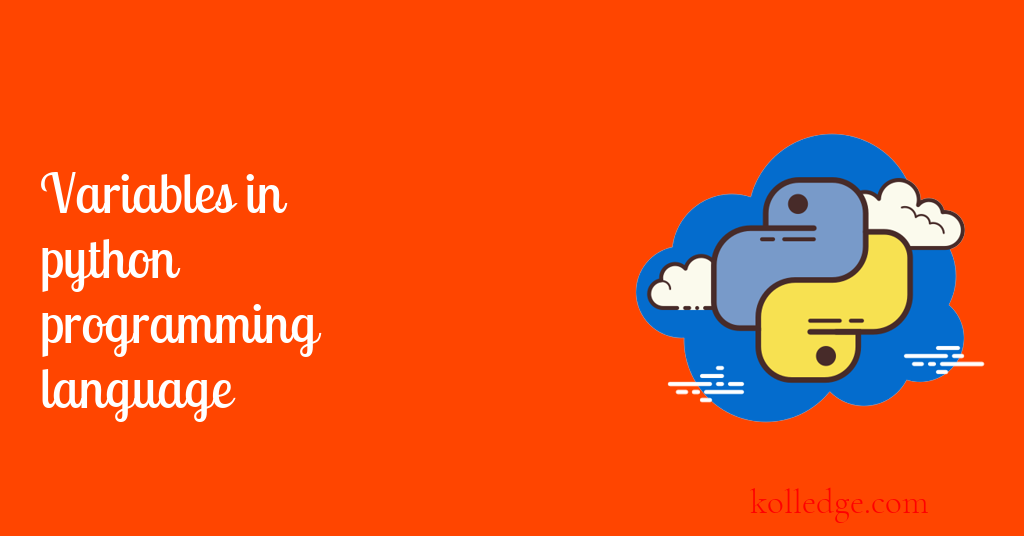
Table of Contents :
- What is a Variable
- Naming Variables in Python
- Data Types of Variables
- Changing Data type through reassignment
- Changing Data type through type casting
- Built-in Data Types in Python
- Getting the type of a value
What is a Variable
- A variable is a named location used to store data in the memory of a computer.
- They are used to store information such as numbers, text, and more.
- There is no separate command to declare variables in Python.
- In Python, variables are created when we assign a value to them.
- Here is an example of creating and using a variable in Python:
myvar = 10
print(myvar)
# Output
# 10
- We can also assign multiple variables at once:
x, y, z = 1, 2, 3
print(x)
print(y)
print(z)
# Output
# 1
# 2
# 3
- We can also use the input() function to get input from the user and store it in a variable:
myvar = input("Enter a value: ")
print(myvar)
# Output
# This would prompt the user to enter a value
# and then print whatever they entered.
Naming Variables in Python
- Python follows strict rules and naming conventions.
- Variable names can be made up of
- letters,
- numbers, and
- underscore characters.
- They cannot start with a number though.
- Python is case sensitive, so the variables
My_var
andmy_var
are not the same. - Code Sample :
My_var = 10
my_var = 20
print("Value of My_var : ", My_var)
print("Value of my_var : ", my_var)
# Output
# Value of My_var : 10
# Value of my_var : 20
Data Types of Variables
- Data Type of a variable specifies the type of data we can store in a variable.
- For Example :
- a variable with int data type can be used to store integers.
- a variable with string data type can be used to store strings of characters.
- In Python Data types are actually implemented as classes.
- The variables that we declare in our code are actually instances of these classes.
- Python is a dynamically typed language, this means that -
- variable's data type need not be declared explicitly at the time of declaration of the variable.
- variable's data type is determined at runtime.
- variable's data type is determined by the type of the value assigned to it.
- variable's data type can be changed simply by reassigning it with a value of some different type.
- variable's data type can also be changed through type casting.
Changing Data type through reassignment :
- The data type of a variable changes if we assign a value of some different data type.
- Below is an example of such type conversion -
x = 100
print("Type of x : ", type(x))
x = 100.2
print("Type of x : ", type(x))
y = 2.0
print("Type of y : ", type(y))
y = 5
print("Type of y : ", type(y))
# Output
# Type of x : <class 'int'>
# Type of x : <class 'float'>
# Type of y : <class 'float'>
# Type of y : <class 'int'>
Changing Data type through type casting :
- Type casting is the method of explicitly specifying the data type of any variable.
- Below is an example of type casting in python -
x = 100
print("Type of x : ", type(x))
x = float(x)
print("Type of x : ", type(x))
y = 2.0
print("Type of y : ", type(y))
y = int(y)
print("Type of y : ", type(y))
# Output
# Type of x : <class 'int'>
# Type of x : <class 'float'>
# Type of y : <class 'float'>
# Type of y : <class 'int'>
Built-in Data Types in Python
Following are the built-in data types available in Python
- Numeric Types
- int
- float
- complex
- Sequence type
- list
- tuple
- range
- Text Sequence Type
- str
- Binary Sequence Types
- bytes
- bytearray
- memoryview
- Set Types
- set
- frozenset
- Mapping Type:
- dict
- Boolean Type
- bool
- None Type
- None
Getting the type of a value
We can use the type(value) function to get the type of a value
t = type(100)
print(t)
t = type(2.0)
print(t)
t = type('Hello')
print(t)
t = type(True)
print(t)
# Output
# <class 'int'>
# <class 'float'>
# <class 'str'>
# <class 'bool'>