Beginner Level
Intermediate Level
Advanced Level
Introduction
Regular expressions, or regex, are an essential tool for data validation and manipulation, and understanding non-capturing groups can greatly enhance your abilities as a Python programmer. Non-capturing groups are an alternative to capturing groups in regex. Unlike capturing groups, they do not store the match to a separate group. This tutorial will explain what non-capturing groups are, when to use them, how to define them, and some practical examples of their usefulness.
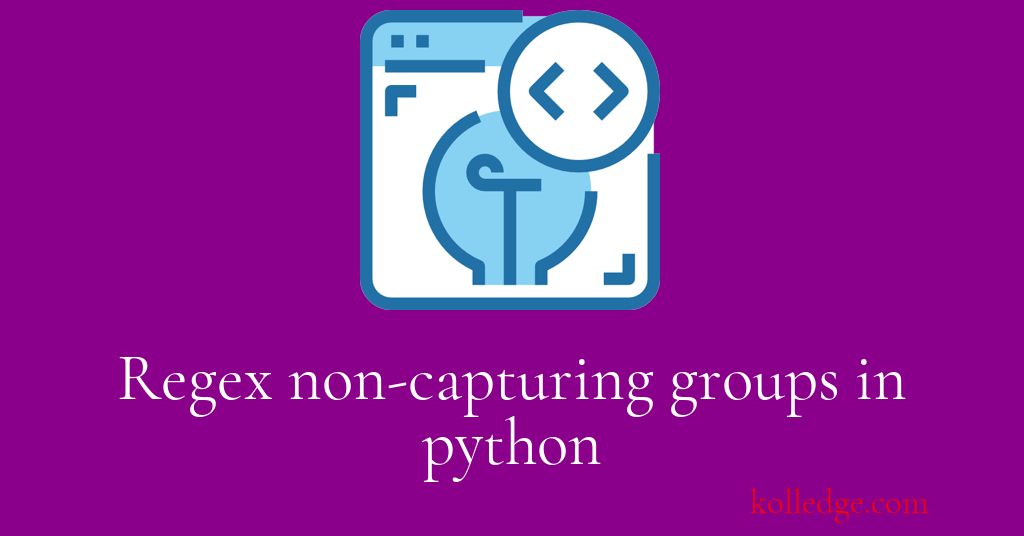
Table of Contents :
- Python Regex Non-capturing Group
- Uses of Python Regex Non-Capturing Group
Python Regex Non-capturing Group :
- Non-capturing group is a feature of Python's regular expression syntax that allows you to specify a parenthesized group that doesn't capture its match.
- Non-capturing group is denoted by the
?:
sequence, which follows the opening parenthesis of the group. - Code Sample :
import re
# Non-capturing group example
text = "The quick brown fox jumps over the lazy dog."
pattern = r"(?:quick|lazy)\s(\w+)"
result = re.findall(pattern, text)
print(result)
# Output:
['brown', 'dog']
Uses of Python Regex Non-Capturing Group :
- Non-capturing group can be useful in situations where you want to match a group, but you don't need to extract its contents.
- For example, suppose you want to search for the words "quick" or "lazy", followed by a space, and then followed by any word character sequence. You can use non-capturing group to achieve this without capturing the first group.
- Code Sample :
import re
# Non-capturing group example
text = "The quick brown fox jumps over the lazy dog."
pattern = r"(?:quick|lazy)\s(\w+)"
result = re.findall(pattern, text)
print(result)
# Output:
['brown', 'dog']
Prev. Tutorial : Alternation
Next Tutorial : Lookahead