Beginner Level
Intermediate Level
Advanced Level
Introduction
In asynchronous programming, it is often necessary to cancel tasks that are no longer needed or that are taking too long to complete. Canceling tasks in Python requires a good understanding of asynchronous programming concepts and techniques, as well as knowledge of the built-in asyncio module and other related libraries. In this tutorial, we will explore the basics of canceling tasks in Python, including the different ways to cancel tasks, handling exceptions, and best practices to follow.
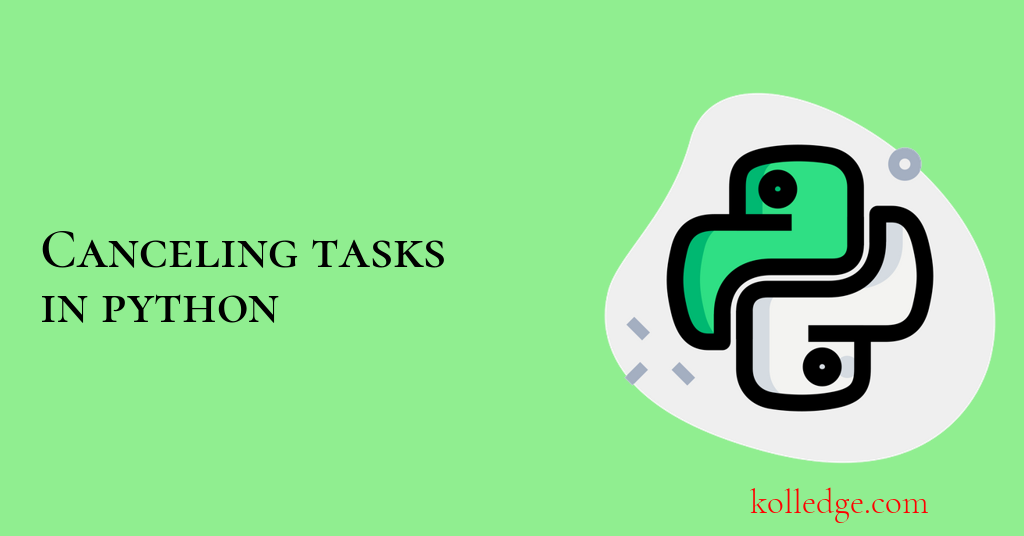
Table of Contents :
- Creating a Long-Running Task
- How to cancel an Asynchronous Task in python
Creating a Long-Running Task :
- In order to demonstrate how to cancel a long-running asynchronous task, we need to create a long-running task first.
- Here's an example of a co-routine that waits for five seconds before returning
- Code Sample :
async def long_operation():
print('Long operation started')
await asyncio.sleep(5)
print('Long operation completed')
return 'Long operation result'
How to cancel an Asynchronous Task in python :
- We can cancel an asynchronous task using the
cancel()
method of the task object. - Here's an example of how to create a task for our
long_operation()
co-routine and then cancel it after three seconds : - Code Sample :
import asyncio
async def long_operation():
print('Long operation started')
await asyncio.sleep(5)
print('Long operation completed')
return 'Long operation result'
async def main():
print('Main started')
long_op_task = asyncio.create_task(long_operation())
await asyncio.sleep(3)
long_op_task.cancel()
print('Long operation cancelled')
try:
await long_op_task
except asyncio.CancelledError:
print('Long operation was cancelled')
else:
print('Long operation result:', long_op_task.result())
print('Main completed')
asyncio.run(main())
Explanation :
- In the above example, we define a co-routine,
long_operation()
, that waits for five seconds before returning. - We also define a
main()
co-routine that creates a task forlong_operation()
and then cancels it after three seconds using thecancel()
method of the task object. - We use a try/except block to catch the
CancelledError
that is raised when the task is cancelled. - When we run this program, it will execute the
long_operation()
co-routine in the background, but cancel it after three seconds. - The program will then print "Long operation cancelled" and "Long operation was cancelled".
Prev. Tutorial : Creating tasks
Next Tutorial : wait_for() function