Introduction
One of the most fundamental concepts in programming is assigning values to variables, and Python provides several assignment operators to make this task easier and more efficient. Assignment operators are used to assign values to variables in different ways, such as incrementing or decrementing their values, or updating them with the result of an arithmetic or logical operation. This tutorial will cover the different types of assignment operators in Python and how they can be used in your programs. Through hands-on examples and explanations, you will gain a solid understanding of this essential aspect of Python programming.
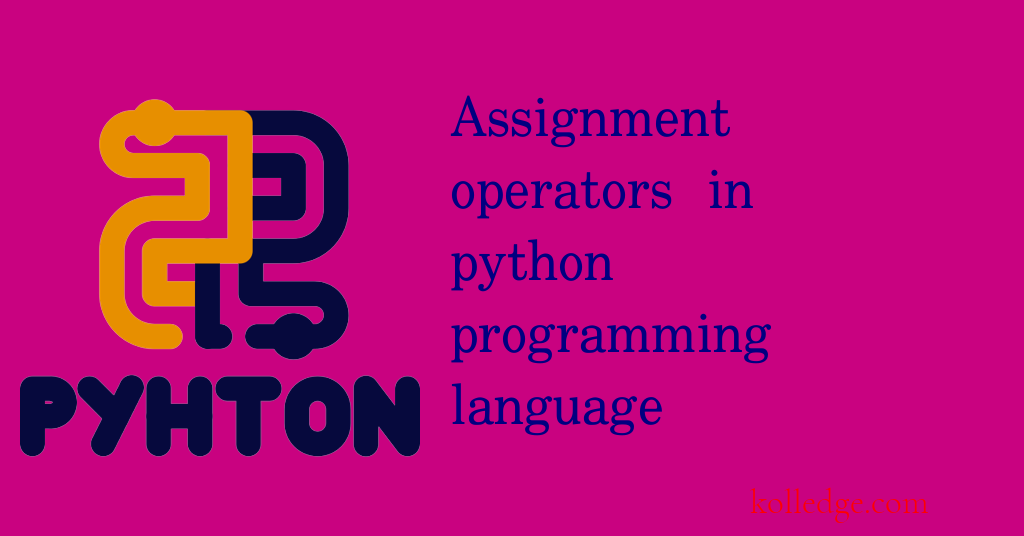
Table of Contents :
- What is Assignment operator
- Shorthand assignment operators in Python
- Types of Shorthand assignment operators in Python
- Add and assign '+='
- Subtract and assign '-='
- Multiply and assign '*='
- Divide and assign '/='
- Modulus and assign '%='
- Exponentiation and assign '**='
- Floor-divide and assign '//='
What is Assignment operator
- Assignment operators are used to assign value to a variable.
- Assignment operator is denoted by equal to sign '='.
- For example
index = 20
assigns an integer value20
to a variable namedindex
. - Code Sample :
x = 100
print(x)
# Output
# 100
Shorthand assignment operators in Python
- Python supports shorthand assignment operators as well.
- Shorthand assignment operators are formed by combining assignment operator with some arithmetic operator.
- Shorthand assignment operators can be used to shorten a long assignment expression
- For example,
x += 2
which is equivalent tox = x + 2
.
Types of Shorthand assignment operators in Python
- There are seven shorthand assignment operators in Python
- Add and assign '+='
- Subtract and assign '-='
- Multiply and assign '*='
- Divide and assign '/='
- Modulus and assign '%='
- Exponentiation and assign '**='
- Floor-divide and assign '//='
Add and assign '+='
- shorthand notation : x += 10
- equivalent expression : x = x + 10
- evaluation: Add 10 to x and assign the new value back to x
- Code Sample :
x = 20
x += 10
print(x)
# Output
# 30
Subtract and assign '-='
- shorthand notation : x -= 10
- equivalent expression : x = x - 10
- evaluation: Subtract 10 from x and assign the new value back to x
- Code Sample :
x = 20
x -= 10
print(x)
# Output
# 10
Multiply and assign '*='
- shorthand notation : x *= 10
- equivalent expression : x = x * 10
- evaluation: Multiply x by 10 and assign the new value back to x
- Code Sample :
x = 20
x *= 10
print(x)
# Output
# 200
Divide and assign '/='
- shorthand notation : x /= 10
- equivalent expression : x = x / 10
- evaluation: Divide x by 10 and assign the new value back to x
- Code Sample :
x = 20
x /= 10
print(x)
# Output
# 2.0
Modulus and assign '%='
- shorthand notation : x %= 10
- equivalent expression : x = x % 10
- evaluation: Perform modulus on the two operands and assign the result to x
- Code Sample :
x = 20
x %= 8
print(x)
# Output
# 4
Exponentiation and assign '**='
- shorthand notation : x **= 2
- equivalent expression : x = x ** 2
- evaluation: Raise x to the power 2 and assign the new value back to x
- Code Sample :
x = 10
x **= 2
print(x)
# Output
# 100
Floor-divide and assign '//='
- shorthand notation : x //= 10
- equivalent expression : x = x // 10
- evaluation: Floor-divide x by 10 and assign the new value back to x
- Code Sample :
x = 20
x //= 8
print(x)
# Output
# 2
Prev. Tutorial : Arithmetic operators
Next Tutorial : Relational operators